Make Your Godot 2D Games Run Smoother Than Ever
Ever felt that frustrating stutter in your game? You are not alone! Optimizing 2D games ensures players have a fantastic experience.
This guide dives into practical ways boost your Godot game’s speed focusing on rendering scripting resource management and avoiding common pitfalls. Many developers share tips online, for instance, discussing optimizing Godot in 2D on Reddit. Let’s make your game fly! 🚀
Key Takeaways:
- Render Smarter, Not Harder: Only draw what players see using tools like VisibilityNotifier2D. Consider baked lighting for static scenes. Address general 2D rendering performance topics.
- Script Efficiently: Use static typing in GDScript, minimize logic in
_process()
, and leverage object pooling systems for repeated items. You might find visual examples helpful. - Manage Resources Wisely: Optimize textures, simplify physics colliders, and streamline assets removing unused elements. Think about efficient loading strategies.
- Profile Your Game: Regularly use Godot’s profiler identify performance bottlenecks early and often. Don’t guess where the lag comes from!
- Avoid Common Mistakes: Watch out for too many nodes, complex particle effects, heavy physics calculations, and unoptimized AI logic. Review common troubleshooting tips.
# Bad practice: Checking time condition every frame
# var time_elapsed = 0.0
# func _process(delta):
# time_elapsed += delta
# if time_elapsed > 5.0:
# print("5 seconds passed!")
# perform_infrequent_action()
# time_elapsed = 0.0 # Reset
# Better practice: Using a Timer node
func _ready():
$ActionTimer.wait_time = 5.0
$ActionTimer.timeout.connect(perform_infrequent_action)
$ActionTimer.start()
func perform_infrequent_action():
print("Timer timed out! Performing action.")
# Reset timer if it should repeat (or set One Shot = false in editor)
# $ActionTimer.start()
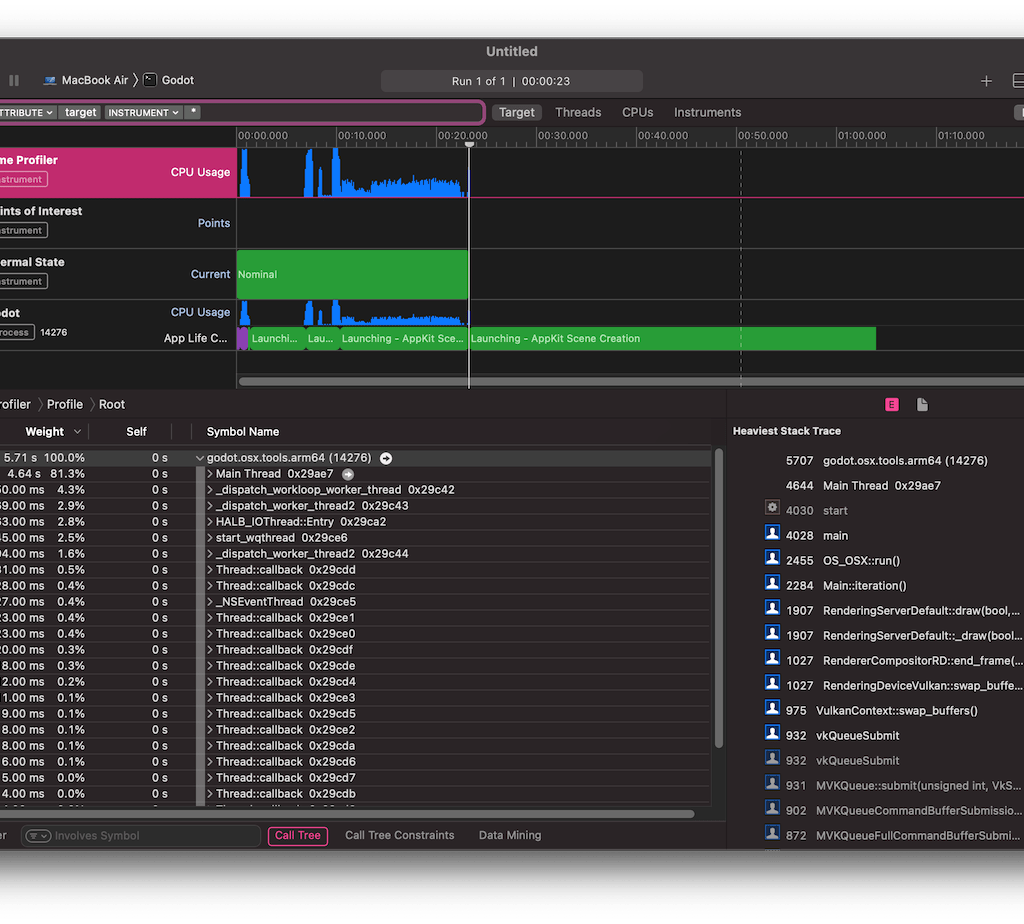
Why Bother With Optimization? 🤔
Slow games lose players FAST. Optimization directly impacts player retention enjoyment. A smooth-running game feels professional polished. It respects the player’s hardware especially on lower-end devices or mobile platforms, a common concern seen in mobile game performance discussions. Good performance can be the difference between a hit game and one that gets uninstalled quickly. Think about it: would you keep playing a game that constantly lags? Probably not, as noted in threads about Godot’s laggy issues. Efficient games just feel better. Some resources even compare different engines for 2D development highlighting the importance of leveraging specific engine features.
Cracking the Code: Rendering Optimization
Rendering often represents a significant part of a game’s workload. Smart rendering choices dramatically improve frame rates.
See Only What Matters: VisibilityNotifier2D
Why render things the player cannot even see? Use the VisibilityNotifier2D
node. Attach this node your objects. It cleverly ensures objects only render when they enter the camera’s view. This simple step reduces unnecessary drawing operations a big win for performance especially in large levels.
# Script attached to the Enemy node which is a parent of VisibilityNotifier2D
func _ready():
# Ensure the notifier node path is correct
var notifier = $VisibilityNotifier2D
if notifier:
notifier.screen_entered.connect(_on_screen_entered)
notifier.screen_exited.connect(_on_screen_exited)
# Initially hide processing if it starts off-screen
set_process(false)
visible = false
func _on_screen_entered():
# Start processing and make visible when entering the screen
print("Enemy entered screen")
set_process(true)
visible = true
func _on_screen_exited():
# Stop processing and hide when exiting the screen
print("Enemy exited screen")
set_process(false)
visible = false
func _process(delta):
# Enemy logic here - only runs when visible on screen
pass

Taming the Particle Storm
Particle effects look cool BUT they can devour resources. Too many particles especially complex ones strain both CPU and GPU.
- Reduce Quantity: Can you achieve a similar visual effect with fewer particles? Try it!
- Sprite Sheet Animations: Consider replacing some particle effects with efficient sprite sheet animations. These often provide good visuals with lower overhead. Unoptimized particles are a known performance drain.
Light It Up Efficiently: Baked vs. Dynamic Lighting
Dynamic lighting adds realism calculating light and shadows constantly. This constant calculation however can be very heavy on the GPU.
- Baked Lighting: For scenes where lighting does not change much bake the lighting. This pre-calculates lighting information into textures significantly reducing real-time GPU load. It is a fantastic trade-off for static environments. Some tutorials might demonstrate lighting techniques.
- Fake It: Sometimes clever visual tricks can simulate lighting effects without the performance cost. Relying heavily on dynamic lights can slow things down.
Texture Tactics: Size and Format Matter
Large unoptimized textures consume lots of memory increase loading times. This particularly impacts performance on devices with limited resources.
- Resize: Ensure textures are not larger than needed for their appearance on screen.
- Compression: Use appropriate texture compression formats supported by Godot manage memory usage effectively. Check Godot’s official performance documentation index for guidance on texture optimization and more.
Multiplying Objects: MultiMeshInstance2D
Need draw many identical objects like coins trees or background details? Drawing each one individually can be slow. Use MultiMeshInstance2D
instead. This node renders large numbers of identical meshes very efficiently often using hardware instancing drastically cutting down draw calls. Visual guides might explain how to implement this.
Shader Strategy
Shaders unlock powerful visual effects. However complex or numerous shaders add significant computational cost. Be mindful of shader usage especially targeting mobile or web platforms like discussed in posts about GLES3 lag. Optimize shader code use simpler effects where possible.
Shadows: Use Sparingly
Real-time shadows look great but they are performance killers.
- Turn Off: Disable shadows completely if they aren’t essential gameplay or aesthetic.
- Shorten Range: If shadows are necessary limit their draw distance. Shorter shadow ranges reduce calculation demands. Heavy shadow use is often cited as a performance issue.
Streamlining Your Scripts: Scripting Optimization
Inefficient code can bog down your game’s CPU performance. Let’s look at GDScript best practices.
The Power of Static Typing
GDScript allows both dynamic static typing. While dynamic typing offers flexibility static typing generally results in faster execution better memory management. Explicitly defining variable types helps Godot optimize code paths. It is a simple change with noticeable benefits.
# Instead of dynamic typing:
# var speed = 150
# var health = 100
# var player_name = "Hero"
# Use static typing:
var speed: float = 150.0
var health: int = 100
var player_name: String = "Hero"
var target_node: Node2D = get_node("SomeOtherNode")
func calculate_damage(amount: float) -> float:
var resulting_health: float = health - amount
health = int(resulting_health) # Cast back if needed
if health < 0:
health = 0
print("Damage calculated, health now: ", health)
return resulting_health # Return type declared
Controlling the Flow: _process()
and _physics_process()
The _process(delta)
_physics_process(delta)
functions run every single frame or physics frame respectively. Placing heavy complex logic here causes constant CPU strain.
WHAT?! You mean I shouldn’t put my entire game logic in _process
?
Exactly!
- Minimize Logic: Keep code inside these functions as lean as possible. Perform only essential updates each frame.
- Event-Driven Approach: Use signals timers or
_input()
events trigger logic instead of checking conditions every frame. For example connect a button’s “pressed” signal run code instead of checkingif Input.is_action_just_pressed("ui_accept"):
inside_process
. - Disable When Idle: If a node or its script is not needed temporarily disable it. This stops its
_process
or_physics_process
functions from running saving resources.
Recycle and Reuse: Object Pooling
Creating destroying objects frequently (like bullets enemies or temporary effects) incurs overhead. Instantiating nodes takes time allocating memory then freeing it later (garbage collection) adds pauses. Object pooling fixes this.
- How it Works: Instead of destroying objects hide them deactivate them. When a new object needed grab an inactive one from the “pool” reset its state make it active again.
- Benefits: Reduces instantiation cost minimizes garbage collection stutters leading smoother gameplay. Search online forums like the Godot Reddit community or the official Godot forums for pooling implementation examples perhaps even video tutorials.
# ObjectPoolManager.gd (AutoLoad/Singleton recommended)
var bullet_scene: PackedScene = preload("res://bullet.tscn")
var bullet_pool: Array[Node2D] = []
const POOL_SIZE = 20
func _ready():
# Pre-populate the pool
for i in range(POOL_SIZE):
var bullet = bullet_scene.instantiate()
bullet.visible = false # Initially inactive
# Add to a specific node in the scene tree to manage them
get_node("/root/GameScene/Bullets").add_child(bullet)
bullet_pool.append(bullet)
func get_bullet() -> Node2D:
for bullet in bullet_pool:
if not bullet.visible: # Find an inactive bullet
bullet.visible = true
# Call a setup function on the bullet script if needed
# bullet.setup(position, direction)
return bullet
# Optional: Pool exhausted, create a new one (or handle error)
print("Bullet pool exhausted! Consider increasing POOL_SIZE.")
var new_bullet = bullet_scene.instantiate()
get_node("/root/GameScene/Bullets").add_child(new_bullet)
bullet_pool.append(new_bullet) # Add to pool for future reuse
return new_bullet
func release_bullet(bullet: Node2D):
# Instead of queue_free(), hide and return to pool
bullet.visible = false
# Reset bullet state if necessary (e.g., position, velocity)
# bullet.position = Vector2.ZERO
Using the pool:
# Player.gd or Weapon.gd
func _shoot():
var bullet = ObjectPoolManager.get_bullet()
if bullet:
# Configure the bullet (position, direction etc.)
bullet.global_position = $Muzzle.global_position
# Assume bullet script has an init or setup function
bullet.initialize_bullet(global_rotation)
# Bullet.gd (When bullet should be removed)
func _on_hit_something_or_lifetime_end():
ObjectPoolManager.release_bullet(self) # Return to pool
# NOT queue_free()
Smart Pathfinding for Crowds
Calculating paths for many AI entities simultaneously can cripple CPU performance. If you have numerous enemies needing navigate:
- Group Calculations: Can nearby enemies share pathfinding results? Perhaps a squad leader calculates path rest follow relatively.
- Stagger Updates: Do all enemies need recalculate their path every single frame? Probably not. Stagger pathfinding updates over several frames distribute the load. Update a few enemies this frame a few next frame so on. Unoptimized navigation requests are a known issue. Complex AI logic without optimization is a common mistake. You might find related discussions or examples in video format.
Watch Out for Nested Loops
Loops are necessary but deeply nested loops (a loop inside another loop inside another…) multiply computation quickly. Review your code: can nested loops be rewritten more efficiently? Sometimes a different data structure or algorithm avoids excessive looping.
Signal Management
Signals are powerful Godot feature but misuse causes bottlenecks. Connecting many signals complex functions or having signals trigger lengthy chains of events impacts performance. Profile signal connections ensure they are not causing unexpected slowdowns. You might encounter discussions around engine weaknesses or specific scenarios like this Reddit thread.
Getting Physical: Physics Optimization
Physics simulation adds realism but requires careful handling avoid performance hits.
Tick-Tock: Physics Tick Rate
Godot runs physics calculations at a fixed rate separate from rendering frame rate. If your game doesn’t need hyper-accurate physics simulation consider lowering the physics tick rate (frames per second) in project settings. Fewer physics steps per second mean less CPU work. Test carefully ensure gameplay doesn’t feel jerky unresponsive.
Keep Colliders Simple
Complex collision shapes require more complex calculations determine overlaps.
- Prefer Primitives: Use simple shapes like rectangles circles or capsules whenever possible instead of detailed polygons.
- Fewer Shapes: Can you represent an object’s collision with one two simple shapes instead of many small ones? Simplify! Every calculation saved helps.
Limit Physics Queries
Constantly querying physics engine (e.g. raycasts shape casts checking for collisions in script) adds load. Minimize unnecessary physics queries. Can checks be done less frequently or only when needed? Excessive physics queries are a common mistake. Sometimes performance issues manifest as jitter which could be related physics or rendering as described in GitHub issues like #82238.
Tidy Up Your Project: Resource Management
A clean well-managed project loads faster uses less memory.
Streamline Your Assets
Over time projects accumulate unused stuff. Regularly prune:
- Unused Scripts Nodes Assets: Delete anything not actually used in your game. This reduces project size clutter.
- Optimize Asset Sizes: We mentioned textures but this applies all assets. Ensure models sounds etc. are appropriately sized compressed. Using large unoptimized assets is a mistake.
Load Smart Not Hard
Loading large scenes or many assets at once causes noticeable pauses or hitches.
- Background Loading: Use Godot’s features load resources or scenes in background threads. This lets the game continue running while assets load preventing freezes.
- Loading Screens: Implement loading screens hide asset loading provide visual feedback player.
- Strategic Loading: Load only what needed for the current game area or level. Unload assets no longer required.
var loader = null
var load_path = "res://large_level.tscn"
var load_progress = 0.0
func start_background_load():
loader = ResourceLoader.load_threaded_request(load_path)
if loader == null:
print("Error: Could not start threaded load.")
else:
set_process(true) # Start checking progress
func _process(delta):
if loader != null:
var status = ResourceLoader.load_threaded_get_status(load_path, progress)
# 'progress' is an array passed to get progress percentage
match status:
ResourceLoader.THREAD_LOAD_IN_PROGRESS:
load_progress = progress[0] * 100.0
# Update a loading bar UI here
print("Loading progress: ", load_progress, "%")
ResourceLoader.THREAD_LOAD_LOADED:
print("Loading complete!")
var resource = ResourceLoader.load_threaded_get(load_path)
if resource:
var new_scene_instance = resource.instantiate()
# Add the loaded scene instance to the tree
get_tree().root.add_child(new_scene_instance)
else:
print("Error: Failed to get loaded resource.")
loader = null # Reset loader
set_process(false) # Stop checking progress
ResourceLoader.THREAD_LOAD_FAILED:
print("Error: Background loading failed.")
loader = null
set_process(false)
ResourceLoader.THREAD_LOAD_INVALID_RESOURCE:
print("Error: Invalid resource path for background load.")
loader = null
set_process(false)
Export Filters Matter
When exporting your game ensure you configure export filters correctly. Files needed at runtime (like JSON config files images loaded dynamically via code) must be included in the export filters otherwise your game might fail load them leading errors or unexpected behavior. Check the general optimization documentation which covers various aspects including export or potentially newer versions like 4.4 or even documentation in other languages if needed.
Avoiding Performance Pitfalls: Common Mistakes
Awareness of common mistakes helps you proactively avoid them.
- Too Many Nodes: Scenes bursting with thousands of nodes even inactive ones increase engine overhead. Keep scene hierarchy organized efficient.
- Unoptimized Particles: As mentioned earlier excessive or complex particles are frequent offenders. Some visual examples might highlight particle system issues.
- Overusing Dynamic Lights/Shadows: Relying too heavily on real-time lighting shadow calculations slows rendering. Bake where possible.
- Inefficient Occlusion Culling: While intended improve performance improper setup of occlusion culling can sometimes cost more than it saves especially in simple scenes. Profile carefully.
- Excessive Physics/AI Calculations: Running too many physics queries or complex AI logic every frame without optimization is a recipe for slowdowns. Centralize stagger these calculations.
- Heavy Logic in
_process()
: Performing complex tasks constant checks in frame-by-frame update functions is a major source of CPU bottlenecks. - Large Assets: Using oversized unoptimized textures images or models wastes memory increases load times.
- Ignoring the Profiler: Flying blind is dangerous! Not using Godot’s built-in profiler means you are guessing about performance issues. Profile often identify true bottlenecks. Failing to use profiling tools is a major oversight. Maybe find profiling tutorials.
- Too Many Navigation Requests: Frequent pathfinding requests from many agents overwhelm the NavigationServer. Optimize AI path requests. Sometimes specific bugs reported on GitHub relate performance like issue #93184.
Banishing Lag: Specific Tips for Smoothness
Lag stutters jitter… these ruin player experience. Let’s focus on direct lag reduction techniques discussed in places like this Reddit thread on reducing lag. Many overlap with general optimization but are worth reiterating.
V-Sync: Friend or Foe?
Vertical Sync (V-Sync) synchronizes game’s frame rate monitor’s refresh rate preventing screen tearing. However it can introduce input lag limit frame rate.
- Disable (Carefully): Turning V-Sync off can increase FPS potentially reducing perceived lag BUT it may cause ugly screen tearing. Test this trade-off.
- Double vs Triple Buffering: If using V-Sync double-buffered V-Sync generally offers lower input latency than triple-buffered V-Sync. Experiment settings found in Godot’s documentation on jitter and stutter. Some engine issues like #75830 on GitHub might touch on related rendering or timing problems.
- Adaptive Sync (G-Sync/FreeSync): If player hardware supports it enabling Adaptive Sync technologies provides benefits of V-Sync (no tearing) without fixed frame rate cap offering smoother variable frame rate experience.
Cap Your Frame Rate
Does your game need run at 200 FPS? Probably not. Limiting frame rate (e.g. 60 FPS or even 30 FPS for certain game types or low-end targets) provides more consistent performance prevents unnecessary high resource usage. This avoids wild FPS fluctuations which feel like lag. Set this in project settings possibly following a guide on optimizing project settings.
Leaner Builds: Exclude Unused Modules
When exporting your final game Godot allows excluding engine modules you don’t use (e.g. if you don’t use 3D physics networking etc.). This results in a smaller leaner executable potentially improving load times reducing memory footprint slightly. Check export options carefully.
Stop Debug Printing!
Leaving print()
statements or similar debug output active in release builds significantly slows down game execution. Remember remove all debug prints before exporting final game!
The Indispensable Tool: The Profiler 📊
You cannot effectively optimize what you cannot measure. Godot includes powerful built-in profiler.
- What it Shows: The profiler monitors frame times physics times script function times memory usage draw calls much more. It helps pinpoint exactly what causing slowdowns. You might find demos of profiler usage.
- How Use It: Run your game from editor enable profiler. Interact with game especially in areas where lag occurs. Analyze graphs data identify spikes bottlenecks.
- Regular Use: Don’t wait until end development profile. Profile regularly catch performance regressions early. Make it part of your workflow! Discussions on forums like Godot Forums highlighting performance optimizations or specific user experiences with performance issues often highlight importance profiling.
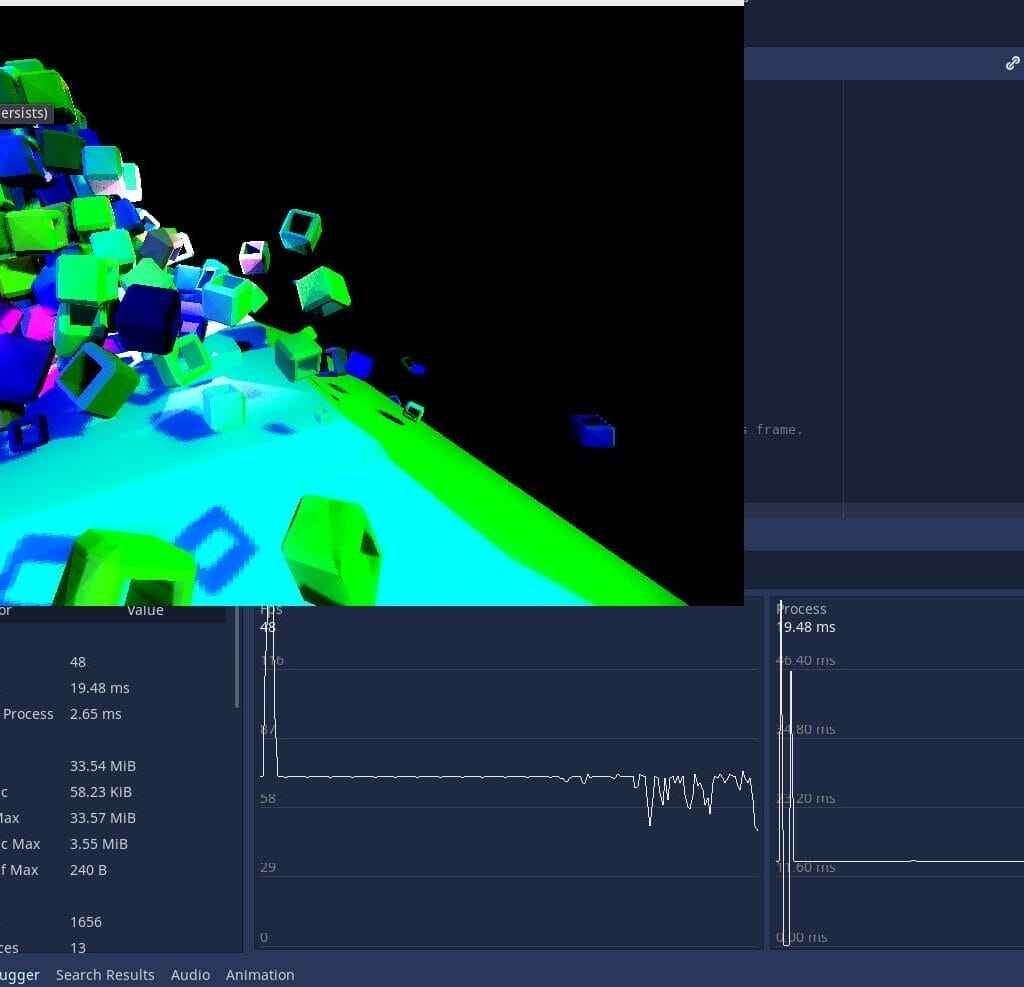
Common Bottlenecks and Solutions
Bottleneck | Solution |
---|---|
Too many active nodes | Pool objects, disable off-screen processing |
Heavy particle effects | Reduce count, use sprite sheets |
Expensive physics | Lower tick rate, fewer colliders, batch AI |
Large textures | Use compressed or smaller assets |
Unused scripts/assets | Remove or disable |
Redundant draw calls | Batch by material/texture |
Optimization Checklist
- [ ] Profile your game regularly
- [ ] Use visibility notifiers for all moving objects
- [ ] Pool and reuse objects
- [ ] Limit physics and script processing
- [ ] Cull off-screen and occluded objects
- [ ] Optimize textures and particles
- [ ] Cap FPS and adjust project settings
Advanced Tips
- Multithreading: Offload heavy calculations to separate threads.
- Native Code: For CPU-intensive logic, consider using C++, C#, or Rust via Godot’s GDExtension system.
- Compute Shaders: For advanced effects, leverage GPU compute shaders.
Conclusion: The Optimization Journey
Optimizing your Godot 2D game is an ongoing process not a one-time fix. It involves understanding how different engine parts interact making smart choices about rendering scripting physics resource management. Start with low-hanging fruit like VisibilityNotifiers simple colliders. Use the profiler religiously guide your efforts. Avoid common mistakes be mindful resource usage. By applying these techniques found across various community discussions official documentation and guides you can create games that run smoothly delight players regardless their hardware. Happy optimizing! ✨
FAQ Godot 2D Game Optimization Secrets in Godot Engine
What is the introduction to optimizing 2D games in Godot Engine?
A: The introduction to optimizing 2D games in Godot Engine focuses on techniques to enhance performance, ensuring that your game runs fast enough even with intensive calculations and a lot of enemies on screen. This involves understanding the Godot version you are using and applying best practices in game development.
How can I optimize the performance of my game in Godot Engine?
A: You can optimize the performance of your game by reducing the complexity of your scenes, using efficient nodes, and minimizing the number of draw calls. Additionally, leveraging the Godot documentation in English will provide insights into specific performance optimization techniques relevant to your Godot version.
What role do nodes play in performance optimization?
A: Nodes are fundamental to Godot’s scene system. Choosing the right node type and structure can significantly affect your game’s performance. For example, using fewer but more complex nodes can be more efficient than a lot of simpler nodes.
How does GDScript affect game performance in Godot?
A: GDScript can impact performance depending on how it’s used. Writing efficient GDScript code, avoiding unnecessary calculations, and using built-in functions can help maintain stable performance. Profiling your GDScript can reveal bottlenecks in your code.
Are there specific geometry considerations for optimizing 2D games?
A: Yes, geometry plays a critical role in optimizing 2D games. Simplifying collision shapes, reducing the number of vertices, and reusing geometry when possible can lead to better performance, especially on devices with integrated GPUs like Intel or Nvidia.
How can I handle a lot of enemies without slowing down my game?
A: To handle a lot of enemies without slowing down your game, consider using object pooling to reuse enemy instances, limiting their visibility, and optimizing their AI calculations. This way, you reduce the overhead associated with creating and destroying instances frequently.
What are some common pitfalls to avoid in Godot performance optimization?
A: Common pitfalls include neglecting to profile your game to identify performance issues, overusing complex shaders or graphics, failing to manage visibility efficiently, and not optimizing node hierarchies. Staying aware of these issues can help keep your game’s performance stable.
How does the Godot version impact optimization techniques?
A: Different Godot versions may offer new features or improvements that can enhance performance. For instance, Godot version 4.2.1 may provide better rendering techniques or optimizations that older versions lack, so always refer to the latest documentation for the best practices.
What is the significance of performance in game development?
A: Performance is crucial in game development as it affects the player’s experience. A game that runs slower or has noticeable lag can lead to frustration. Ensuring a smooth and responsive experience is key to keeping players engaged.