Dive Deep into Dice Rolls, Lottery Picks, and More – And How They Make Your Favorite Games Tick!
🔑 Key Takeaways: Random Number Generators
- Random Number Generators (RNGs) are the secret sauce! They create surprise and replayability in everything from board games to complex video games.
- Simple code, big impact! Basic RNGs like dice rollers or coin flips are easy to create but form the foundation of many game mechanics.
- Customization is king! Tailoring RNGs to specific game needs – like custom ranges for stats or unique lottery draws – is crucial for game design.
- Beyond games! While essential for interactive entertainment, the principles of RNGs pop up in simulations, decision-making, and even art.
- Understanding RNGs empowers you! Whether you’re a budding game developer or just curious, knowing how chance is generated can deepen your appreciation for game design.
Unlocking the Fun: Your Ultimate Guide to Random Number Generators in Games (and Beyond!)

Have you ever wondered what makes your favorite video game so excitingly unpredictable? Or how a board game can feel fresh every time you play? Chances are, a clever little thing called a Random Number Generator, or RNG, is working its magic behind the scenes. RNGs are more than just lines of code; they are the heart of surprise, the engine of replayability, and the artists painting unique experiences every single time. They are the digital equivalent of rolling dice, shuffling a deck of cards, or spinning a prize wheel.
This guide will pull back the curtain on these fascinating tools. We’ll explore different types of RNGs, from the humble dice roller to more complex lottery pickers. We’ll look at how they’re built (with some simple Python code examples to peek at true random number generation!), where they’re used, and how you can even think about using them yourself. It’s not just for game designers either, understanding how randomness is harnessed can make you appreciate the games you play even more. So, get ready to dive into the world of chance, where numbers dance and create endless possibilities! Theres a lot to discover.
The Building Blocks of Chance: Understanding Basic RNGs
At its core, most “randomness” in computers isn’t truly random in the way flipping a perfectly balanced coin in a vacuum might be. Instead, computers often use something called pseudo-random number generators. These are clever algorithms that produce sequences of numbers that look random and pass many statistical tests for randomness, but if you knew the starting point (called a “seed”), you could predict the sequence. For most games and applications, this is perfectly fine and actually very useful, as it allows developers to recreate specific sequences of randomness if needed, for testing.
In the world of Python programming, a popular language for all sortss of projects, the random
module is your best friend for generating these numbers. It’s like a built-in toolkit for all things chance. Let’s explore some fundamental RNGs you might encounter or want to build.
The Classic Cube: All About Dice Rollers 🎲
The dice roller is perhaps the most iconic random number generator. For millennia, humans have used dice – typically six-sided cubes – to introduce chance into games, divination, and decision-making. A digital dice roller simply mimics this action.
How it Works: A standard dice roller generates an integer (a whole number) between 1 and 6, inclusive. Each outcome (1, 2, 3, 4, 5, or 6) should ideally have an equal probability of occurring.
Python Code Example: Here’s a simple way to make a dice roller in Python:
import random
def roll_dice():
return random.randint(1, 6)
# Example usage
# print("You rolled:", roll_dice())
`
C# Code Example:
// Create a Random object to use across all methods
private static Random random = new Random();
// Classic Dice Roller
public static int RollDice()
{
return random.Next(1, 7); // Next(min, max) where max is exclusive
}
This code snippet uses random.randint(1, 6)
which picks a random whole number from 1 up to 6. Simple, yet effective!
Use Cases:
- Board Games: This is the most obvious. Think Monopoly, Yahtzee, or Dungeons & Dragons. Many digital versions of board games use such a function.
- Role-Playing Games (RPGs): Both tabletop and video game RPGs use dice rolls for combat (to hit, damage), skill checks (can you pick the lock?), and many other mechanics.
- Decision-Making Tools: Can’t decide where to eat? Assign numbers to choices and roll a die!
- Educational Purposes: Demonstrating probability in math classes.
Case Story: The Dungeon Master’s Dilemma Alex, a dedicated Dungeon Master (DM) for a weekly Dungeons & Dragons game, often found themself needing quick, unexpected events to spice up the adventure. Instead of pre-planning every tiny detail, Alex wrote a simple Python script with a roll_dice()
function. When the players wandered into a quiet forest, Alex might mentally assign outcomes: 1-2 means a sudden change in weather, 3-4 means they hear a mysterious sound, 5-6 means a surprise encounter. A quick tap on the laptop, and the dice roll guided the story, making it feel more organic and surprising, even for Alex! It saved a lot of time.
Customization: The beauty of digital dice is their flexibility.
- Different Dice Types: Game designers frequently use dice other than the standard six-sided one (d6). There are 4-sided (d4), 8-sided (d8), 10-sided (d10), 12-sided (d12), and 20-sided (d20) dice, among others. You can easily modify the
roll_dice
function:
#Python Example
def roll_any_dice(sides): return random.randint(1, sides) # print("You rolled a d20:", roll_any_dice(20))
C# Example
// Roll any type of dice public static int RollAnyDice(int sides) { return random.Next(1, sides + 1); } - Multiple Dice: Games often require rolling multiple dice, like 2d6 (two six-sided dice and sum the results). This involves calling the function multiple times.
- Modifiers: Sometimes you add or subtract a number from a dice roll, for example,
roll_dice() + 2
.
The humble dice roller, in its many forms, remains a cornerstone of game design due to its simplicity and the immediate understanding most players have of its function.

Your Numbers, Your Rules: Custom Range Generators
What if you need a random number that isn’t tied to a die? Perhaps you need a percentage (1 to 100), or a specific range for an enemy’s health points, or to determine how many gold coins a treasure chest contains. This is where a custom range number generator comes in handy.
How it Works: This type of generator produces a random integer between a minimum value and a maximum value that you define. It’s incredibly versatile.
Python Code Example:
import random
def random_number(min_value, max_value):
return random.randint(min_value, max_value)
# Example usage: random number between 10 and 50
# print("Random number:", random_number(10, 50))
C# Example
// Custom Range Generator
public static int RandomNumber(int minValue, int maxValue)
{
return random.Next(minValue, maxValue + 1);
}
Just like the dice roller, random.randint()
is the star, but here min_value
and max_value
can be any integers you choose (as long as min_value
is not greater than max_value
).
Use Cases:
- Stat Generation in Games: When creating a character in an RPG, stats like strength, agility, or intelligence are often determined by random numbers within a certain range (e.g., 3 to 18).
- Loot Drop Chances: When you defeat an enemy, the chance of it dropping a rare item might be determined by rolling a number between 1 and 1000, which can be influenced by random digits. If the number is, say, below 5, you get the super rare sword!
- Event Triggers: A game might check every few seconds if a random event should occur, influenced by many random numbers. For example,
random_number(1, 100)
could be called, and if it’s a 1, a special merchant appears. - Simulations: Simulating anything from stock market fluctuations (within a range) to the number of customers entering a shop per hour.
- Procedural Content Generation: Creating varied game levels by randomizing dimensions of rooms, number of obstacles, or placement of items within certain boundaries.
Case Story: Sarah’s Indie Game Success Sarah was developing her first indie video game, a charming platformer. She wanted the enemies to feel a bit different each time you encountered them. Instead of giving every “Grublin” (her game’s basic foe) the exact same movement speed and attack power, she used a custom range generator. Each Grublin, when spawned, would get a speed value using random_number(8, 12)
and an attack delay using random_number(1, 3)
seconds. This small touch made the Grublins slightly unpredictable some were a bit faster, others attacked a little quicker. Players loved the subtle variety, and it made the game feel more alive, contributing to its suprising popularity on indie game portals.
Customization:
- Dynamic Ranges: The
min_value
andmax_value
don’t have to be fixed. They could change based on game difficulty, player level, or other factors. - Non-Uniform Distributions (Advanced): While
random.randint()
gives a uniform distribution (each number is equally likely), sometimes game designers want certain numbers to be more common than others. For instance, you might want mid-range stats to be more common than very high or very low stats. This often involves more complex math or different functions from therandom
module (likerandom.triangular()
orrandom.gauss()
), but the core idea of picking from a range remains. This is a bit more advanced, but good to know its possible.
Custom range generators are the workhorses of many game systems, providing flexible randomness wherever it’s needed.
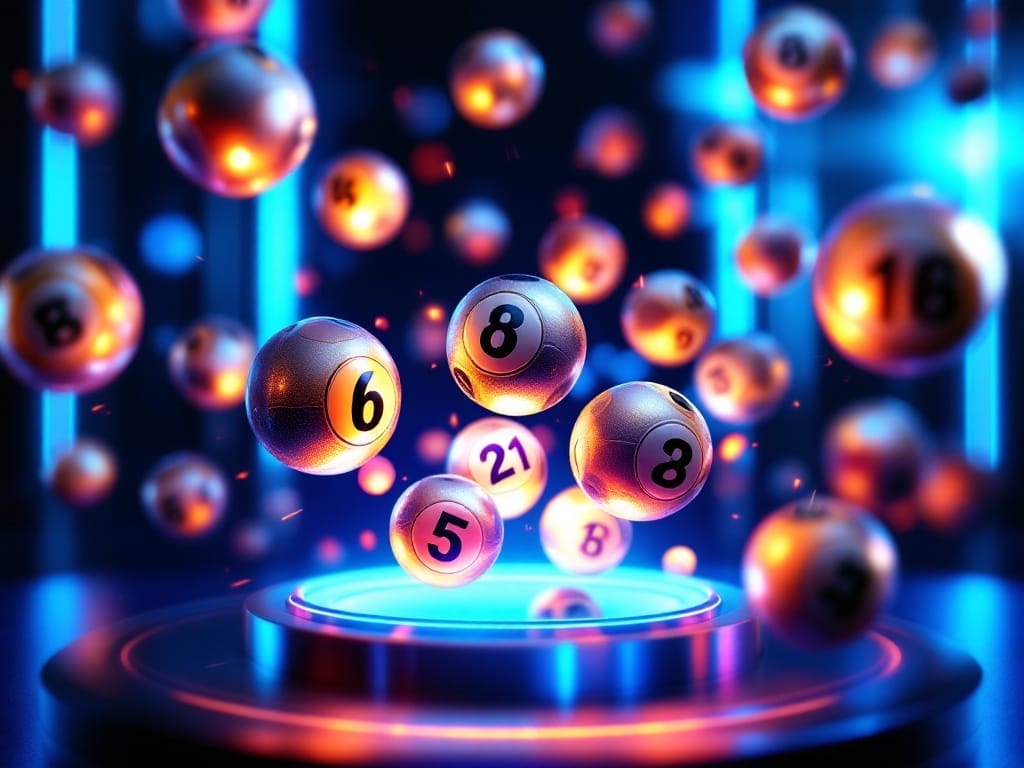
Picking Winners and Flipping Fates: RNGs for Selection
Sometimes, you don’t just need a random number; you need to randomly select one or more items from a group. Think of drawing names from a hat or picking lottery numbers.
The Dream of the Jackpot: Lottery Number Pickers 🎰
A lottery number picker simulates the common game of chance where a few numbers are randomly drawn from a larger set of possible numbers. The key here is usually that once a number is picked, it can’t be picked again in the same draw (it’s unique).
How it Works: You specify how many numbers you want to draw (e.g., 6 numbers) and the range they should come from (e.g., 1 to 49). The generator then randomly selects the specified quantity of unique numbers from that range.
Python Code Example:
import random
def lottery_numbers(draws=6, max_number=49):
# random.sample ensures unique numbers are picked
return sorted(random.sample(range(1, max_number + 1), draws))
# Example usage
# print("Lottery numbers:", lottery_numbers())
C# Code Example:
public static List<int> LotteryNumbers(int draws = 6, int maxNumber = 49)
{
// Create a list of all possible numbers
List<int> numbers = Enumerable.Range(1, maxNumber).ToList();
// Select random numbers without replacement
List<int> result = new List<int>();
for (int i = 0; i < draws && numbers.Count > 0; i++)
{
int index = random.Next(0, numbers.Count);
result.Add(numbers[index]);
numbers.RemoveAt(index);
}
// Sort the results
result.Sort();
return result;
}
Here, random.sample(population, k)
is perfect. It takes a population
(in this case, all numbers from 1 to max_number
) and an integer k
(the number of draws
), and returns a list of k
unique items chosen from the population. We sorted()
them just to make them look nice, like in a real lottery.
Use Cases:
- Actual Lottery Simulations: For fun, or to test theories about lottery outcomes (though remember, each draw is independent!).
- Drawing Winners for a Contest: If you have a list of participants, you can assign them numbers and use this to pick winners fairly.
- Selecting Random Challenges in a Game: A game might have 50 possible mini-challenges, and it picks 3 at random for the player to complete.
- Creating Unique Combinations: Generating unique sets of features for characters, items, or levels. For example, picking 3 unique magical properties out of a list of 20 for a legendary weapon.
- Card Games: Simulating drawing a hand of cards from a deck (where each card is unique).
Case Story: The School Fair Raffle The student council at Northwood High was organizing their annual school fair, and the main event was the grand raffle. They had sold hundreds of tickets, each with a unique number. To ensure fairness and transparency, Maya, the council secretary and a budding coder, suggested using a Python script instead of manually drawing tickets from a large drum. She adapted the lottery_numbers
function. The max_number
was the total number of tickets sold, and draws
was the number of prizes. On fair day, they projected the script onto a big screen, everyone watched as Maya ran it, and the winning numbers were picked instantly and impartially. It was a huge hit, and even the principal was impressed by their innovative approach.
Customization:
- Number of Picks (
draws
): Easily change how many items are selected. - Range of Numbers (
max_number
): Adjust the pool from which numbers are drawn. - Picking from a List of Items:
random.sample
can also pick from a list of non-numeric items, like names or Categoriess.# tasks = ["Quest A", "Quest B", "Quest C", "Quest D", "Quest E"] # chosen_tasks = random.sample(tasks, 2) # print("Your random tasks:", chosen_tasks)
Lottery pickers are great for any situation that requires selecting a subset of unique items from a larger collection, ensuring fairness and unpredictability.
Heads or Tails? The Simple Power of a Coin Flip 🪙
The coin flip is perhaps the simplest form of RNG, representing a binary choice – two possible outcomes, each equally likely.
How it Works: It randomly selects one of two options, typically “Heads” or “Tails.”
Python Code Example:
import random
def coin_flip():
return random.choice(['Heads', 'Tails'])
# Example usage
# print("Coin flip:", coin_flip())
C# Code Example:
// Coin Flip
public static string CoinFlip()
{
return random.Next(2) == 0 ? "Heads" : "Tails";
}
The random.choice()
function is ideal here. You give it a list (or any sequence), and it picks one item randomly from that list.
Use Cases:
- Simple Decision Making: Can’t decide who goes first? Flip a coin!
- Breaking Ties: If two players have the same score, a coin flip can be a fair tie-breaker.
- Determining Turn Order: In some simple games.
- Triggering Binary Events in Games: Should a guard turn left or right? Does a specific rare event happen (50% chance) or not?
- Boolean Flags: In programming, you might set a true/false flag randomly.
Case Story: Two Friends and a Pixelated Argument Liam and Chloe were playing a cooperative online game. They found a single, powerful potion, and both wanted it. Their characters stood there, an impasse. The game, however, had a neat little “/flipcoin” emote that would display “Heads!” or “Tails!” in the chat. “Okay,” Liam typed, “Heads I get it, Tails you get it.” Chloe agreed. Liam typed /flipcoin
. A moment of suspense… “Heads!” The game’s simple coin flip resolved their friendly dispute quickly and fairly, and they got back to adventuring. Even such a tiny RNG feature can smooth out player interactions.
Customization:
- More Than Two Choices: While a coin is binary,
random.choice()
can handle more options easily. You could make a “three-sided coin” or pick from any list of options:# options = ["Attack", "Defend", "Special Move"] # ai_action = random.choice(options) # print("AI chose:", ai_action)
- Weighted Choices (Advanced): Sometimes you don’t want choices to be equal. Maybe “Heads” should appear 70% of the time. This is possible with
random.choices()
(note the ‘s’) which allows you to specify weights, but its a bit more complex than our basic flip.
The coin flip, despite its simplicity, is a fundamental tool for introducing a quick, unbiased 50/50 chance into any system.
More Than Just Numbers: Visual and Interactive RNGs
Sometimes, just seeing a number isn’t as engaging as a more visual representation of chance. That’s where things like spinners come in.
Round and Round It Goes: The Spinner ✨
A spinner, like those found in many board games or prize wheels, is a visual way to make a random selection from a number of fixed segments.
How it Works: You imagine a circle divided into a certain number of equal segments, each typically numbered. The spinner is “spun,” and the segment it lands on is the outcome. Digitally, this is usually simplified to just picking a random number representing one of the segments.
Python Code Example:
import random
def spinner(segments=8):
return random.randint(1, segments)
# Example usage
# print("Spinner landed on:", spinner())
C# Code Example:
// Spinner
public static int Spinner(int segments = 8)
{
return random.Next(1, segments + 1);
}
This is essentially like our custom range generator, where the min_value
is 1 and the max_value
is the number of segments
. If you have an 8-segment spinner, it will return a number from 1 to 8.
Use Cases:
- Virtual Board Games: Many board games (like Twister, or The Game of Life) use spinners. Digital versions need this functionality.
- Prize Wheels in Apps: “Spin to win!” promotions in mobile games or apps often use a visual spinner linked to an RNG like this to determine the prize.
- Mini-Games: A quick spinner mini-game can add variety to a larger game.
- Selecting Categories or Actions: In a trivia game, a spinner might select the question category. In an RPG, it might determine which random buff or debuff a player gets.
Case Story: The Daily Reward Spinner A mobile game company, “PixelPals Inc.,” wanted to improve daily player engagement for their popular pet simulation game. They introduced a “Daily Reward Spinner.” Every day, players could spin a colorful, animated wheel with 8 segments. Segments offered various in-game currencies, rare items, or pet accessories. Behind the animation, a simple spinner(8)
function (with results mapped to prizes) determined the outcome. Player retention went up; people loved the little thrill of the daily spin, and the visual feedback was much more exciting than just being told “You received 100 gold.”
Customization:
- Number of Segments: Easily adjustable. A spinner could have 3 segments or 20.
- Weighting Segments (Advanced) using noise and true random numbers: This is a common and important customization, especially for prize wheels. You might want the “Grand Prize” segment to be much less likely to be hit than the “Small Coin Pouch” segment. This requires more than
random.randint()
. You’d typically assign probabilities to each segment and use a function likerandom.choices()
with aweights
parameter. This makes sure rare items stay rare! - Visual Representation: The code gives you a number. In a real application, you’d link this number to a graphical spinner animation for better player experience.
Spinners offer a more dynamic and often more engaging way to present random choices, especially when a visual element is desired.
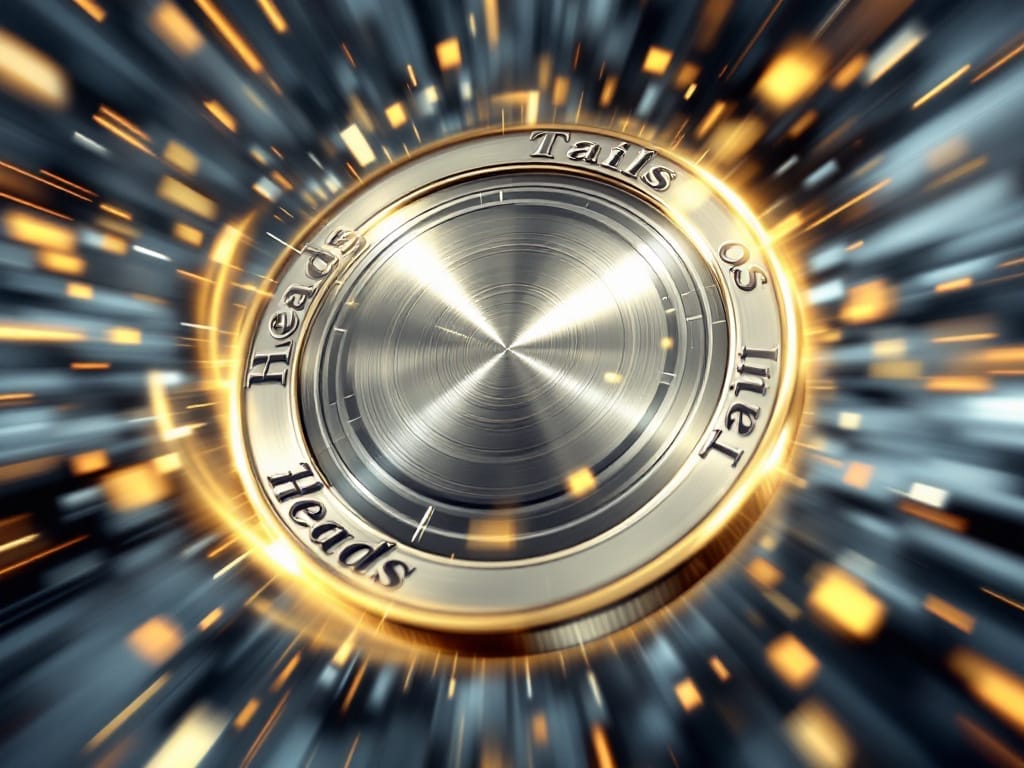
Making RNGs Work For You: Smart Implementation
Having the tools to generate random numbers is one thing; using them effectively is another. How do developers integrate these into games and other applications in a way that’s fun, fair, and functional?
Powering Digital Worlds: RNGs in Video Games
RNGs are practically the lifeblood of many video game genres. Their applications are vast and varied:
- Unpredictability in Enemy AI: Will the enemy patrol left or right? Will they use a special attack now or later? Simple RNGs can make enemy behavior less predictable and more challenging.
- Loot Systems: This is a big one. When you defeat a monster or open a chest, what treasure do you get? The rarity, type, and stats of items are often heavily determined by true random numbers generated. Games like Diablo or Borderlands are famous for their RNG-driven loot.
- Procedural Generation: Many games create levels, maps, or even entire galaxies “on the fly” using algorithms heavily reliant on RNGs. Games like Minecraft or No Man’s Sky use procedural generation to offer virtually limitless unique environments. The RNG decides terrain features, resource placement, creature distribution etc.
- Critical Hits: In combat systems, a “critical hit” (doing extra damage) is often a chance event, like a 10% probability, determined by an RNG roll (e.g., roll 1-100, if 10 or less, it’s a crit!).
- Game Events: Random encounters, weather changes, a surprise merchant appearing – all these can be triggered by RNGs to keep the game world dynamic.
Fairness and Player Perception: A crucial aspect for game developers is how players perceive randomness. True randomness can sometimes produce “streaks” – you might get three critical misses in a row even if the chance is low. This can feel unfair to players. Some games implement “pity timers” or “pseudo-random distribution” systems that adjust probabilities behind the scenes to make outcomes feel more evenly distributed over time, even if it means sacrificing true randomness for a better player experience. For example, if you haven’t gotten a rare item after many tries, the system might subtly increase your chances.
Beyond the Screen: RNGs in Board Games and More
While we’ve seen Python examples, the concepts of these RNGs are everywhere, even without a computer:
- Virtual Companions for Physical Games: Don’t have dice? Many apps provide dice rollers. Need to pick a starting player? A coin flip app will do.
- Educational Tools for Probability: Teachers can use these simple generators (or have students build them!) to demonstrate concepts of chance, probability, and statistics in an interactive way. Imagine simulating thousands of coin flips instantly to show that it tends towards 50/50.
- Quick Decision-Making in Everyday Life: Can’t decide on a movie? Number your choices and use a custom range generator or spinner. It adds a fun, impartial element to mundane choices.
- Brainstorming and Creativity: Stuck for an idea? Create lists of words or concepts and use an RNG to pick a few at random to see if they spark a new connection.
Tailoring the Random: Why Customization Matters
As we’ve seen with each RNG type, customization is key. Simply dropping a generic random.randint(1, 100)
into every situation isn’t good design.
- Game Balance: RNGs must be tuned carefully. If a random weapon drop is too powerful, it can break the game’s difficulty. If random penalties are too frequent, the game becomes frustrating. Developers spend a lot of time tweaking the ranges, probabilities, and types of RNGs to ensure their games are challenging but fair.
- Player Experience: The goal of randomness in games is usually to enhance fun and replayability. Frustrating randomness (e.g., constantly missing an attack with a high chance to hit) can drive players away. The implementation should feel right for the game.
- Theming: The type of RNG can also match a game’s theme. A pirate game might use dice that look like skulls. A sci-fi game might have a futuristic data-stream animation for its “random” picks. The presentation matters.
- Predictability vs. Surprise: Some games want more predictability, using RNGs only for minor variations. Others thrive on wild, unexpected outcomes. The level of randomness is a deliberate design choice.
Good game design involves understanding the mathematical properties of RNGs and, just as importantly, the psychological impact they have on players. It’s an art and a science.
The Wider World of Randomness (A Quick Peek)
While our focus has been on games and simple generators, it’s worth noting that random number generation is a massive field with critical applications far beyond entertainment.
- Scientific Simulations: RNGs are vital for simulating complex systems in physics, biology, finance (e.g., stock market models), climate science (e.g., weather prediction models), and more. These often require extremely high-quality, statistically robust random numbers.
- Cryptography: Secure communication and data protection rely heavily on RNGs to generate encryption keys that are impossible (or computationally infeasible) to guess. The randomness here needs to be top-notch; predictable “random” numbers would be a security disaster.
- Statistical Sampling: When conducting surveys or research, RNGs are used to select representative samples from a larger population, ensuring unbiased results.
- Art and Music Generation: Algorithmic art and music often use RNGs to introduce variation, surprise, and organic-feeling patterns into creative works.
The human fascination with chance is ancient. From oracle bones to lottery tickets to complex video game algorithms, we’ve always found ways to incorporate unpredictability into our lives for fun, fairness, or discovery. These foundational examples of RNGs, similar to ones you might find when exploring resources provided by AI tools and knowledge bases such as those found on platforms like Perplexity and other educational sites, offer a fantastic starting point for anyone looking to understand or implement chance in their own projects. The journey into randomness is a deep one, and these basic building blocks are where it all begins.
Bringing It All Home: Final Thoughts on Randomness
Random Number Generators, from the simplest coin flip to the intricate algorithms powering massive game worlds, are fundamental tools for creating dynamic, engaging, and replayable experiences. They inject surprise, ensure fairness (when designed well!), and can even help us make decisions. The Python examples we’ve looked at show just how accessible creating these mechanisms can be.
Whether you’re a gamer curious about what makes your favorite titles tick, an aspiring developer looking to add some unpredictability to your creations, or just someone interested in the mechanics of chance, understanding RNGs opens up a new perspective. The key is not just in generating a number, but in how that number is used—how it’s woven into the rules, the narrative, and the overall experience.
So, next time you roll a digital die, get a surprise loot drop, or see a virtual wheel spin, remember the elegant simplicity and underlying power of the RNG working behind the screen. Don’t be afraid to experiment with these concepts; the world is full of interesting ways to play with probability. The future of interactive experiences will undoubtedly find even more creative ways to harness the captivating nature of chance!
Frequently Asked Questions (FAQ) Random numbers generators
How do random number generators generate numbers?
A: Random number generators produce random numbers based on algorithms or physical processes, such as atmospheric noise or quantum phenomena, to create a random sequence of different numbers.
Can I customize a random sequence to avoid duplicates?
A: Yes, you can customize a randomizer to generate a list of numbers without duplicates by setting specific parameters to ensure that each number is different.
What is the difference between a truly random sequence and a pseudo-random sequence?
A: A truly random sequence is generated from unpredictable physical processes, like quantum events, while a pseudo-random sequence is produced by algorithms that can eventually repeat the same input, leading to patterns.
How can I generate random numbers within a specific range?
A: To generate random numbers within a specific range, you need to define the minimum and maximum numbers (min and max) for the randomizer to ensure that the output falls within your desired limits.
What methods can I use to generate random numbers?
A: You can use various methods to generate random numbers, including software algorithms, hardware randomizers, or even physical processes like rolling dice or drawing lots.
Is it possible to generate a random sequence of numbers without duplicates?
A: Yes, it is possible to generate a random sequence of numbers without duplicates by using a random number generator that specifically avoids repeating the same number in the output.
How does atmospheric noise contribute to generating random numbers?
A: Atmospheric noise provides a source of entropy that can be harnessed to produce random numbers, creating a truly random sequence that is less predictable than algorithm-based methods.
Can quantum random number generators produce random numbers faster than classical ones?
A: Quantum random number generators can produce random numbers at a faster rate due to their reliance on the inherent unpredictability of quantum mechanics, allowing for the generation of different numbers quickly.
What is the significance of the smallest and largest values in a random sequence?
A: The smallest and largest values in a random sequence define the range of the output, ensuring that the generated numbers fall between these two limits, thus maintaining the desired characteristics of the random numbers.